25 Design widgets
Framework7 brings dozens of different widgets like a photo browser, virtual lists (high performance lists), messages, notifications and toasts. Figure 25.1 shows from left to right the chat widget, the floating action buttons and the gauges.
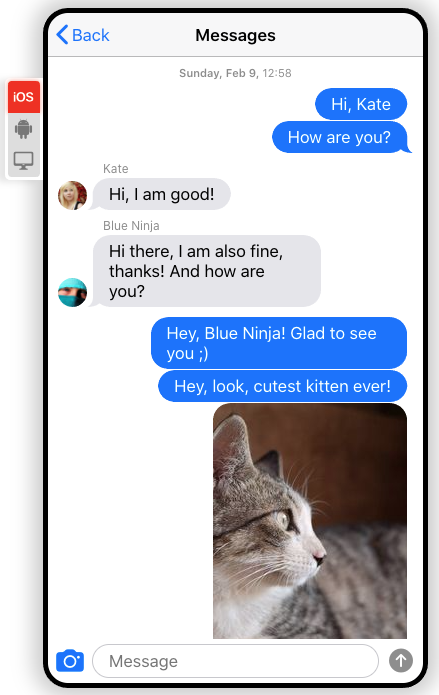
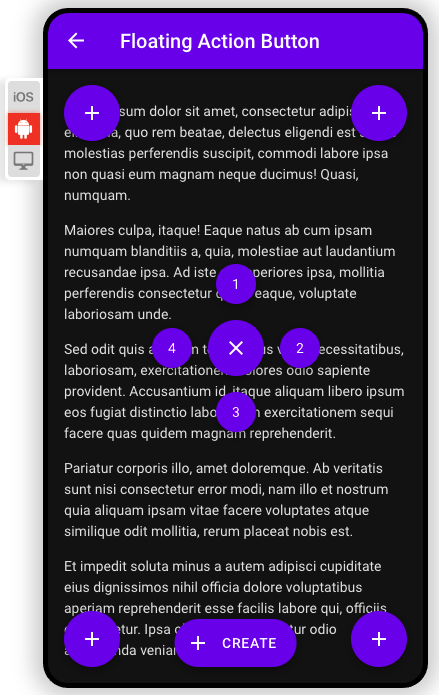
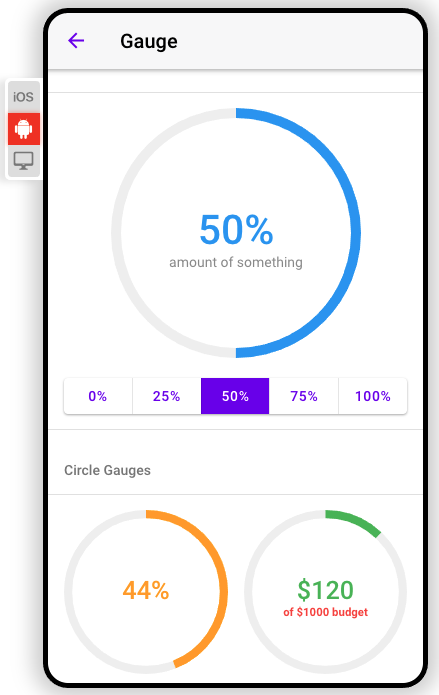
FIGURE 25.1: Framework7 widgets. From left to right: chat, floating action button and gauges.
Looking at the documentation, the API is most of the time always the same, that is, we create the widget:
.widget.create(parameters); app
and we update, open or close it later:
.widget.update(newParameters);
app.widget.open();
app.widget.close(); app
I must admit, there are few deviations like the navbar (app.navbar.show()
) or the modal dialog, but we have enough common points to design a main wrapper that creates any widget and update/open/close it.
What we do below significantly simplifies the R/JS API by providing a general method to initialize and update some of those widgets.
As a reminder, the code examples shown throughout this chapter are gathered in the {OSUICode}
package accessible here: https://github.com/DivadNojnarg/OSUICode/tree/1d42aa7531705954d3c21921c8bbb10623b20d12, specifically at https://github.com/DivadNojnarg/OSUICode/blob/1d42aa7531705954d3c21921c8bbb10623b20d12/R/shinyMobile.R#L151 for widgets.
25.1 Build the UI
We know that JavaScript must receive a configuration object to create the widget instance. As shown earlier in this book, there is a simple way to achieve this. Let’s consider the gauge example:
On the UI side, we expect to have:
<div class="gauge"></div>
Upon widget instantiation, Framework7 populates this container with the relevant tags and attributes. The f7_gauge()
function creates a div
tag with the gauge
class, as well as a configuration tag:
f7_gauge <- function(id, value, options = NULL) {
if (is.null(options[["valueText"]])) {
options[["valueText"]] <- paste(value * 100, "%")
}
gaugeProps <- c(list(value = value), options)
gaugeConfig <- shiny::tags$script(
type = "application/json",
`data-for` = id,
jsonlite::toJSON(
x = gaugeProps,
auto_unbox = TRUE,
json_verbatim = TRUE
)
)
shiny::tags$div(
class = "gauge",
id = id,
gaugeConfig
)
}
We provide a default for the valueText
option that should display the current
value followed by a %
symbol. Note that the class is crucial to target the relevant tag on the JS side. All other widgets more or less follow the same scheme. Be careful about partial matching
with the $
operator. This is the reason why we used [[
instead: with $
, valueText
could be
matched with valueTextColor
, leading to unexpected behavior.
25.2 Widgets without preexisting UI
There are few widgets like toasts and notifications that don’t have any predefined UI element when the app starts. In this case, we simply send the configuration to JS, through the session:
f7_notif <- function(
id = NULL,
text,
options = NULL,
session = shiny::getDefaultReactiveDomain()
) {
if (!is.null(options$icon)) {
options$icon <- as.character(options$icon)
}
message <- c(
dropNulls(list(id = session$ns(id), text = text)),
options
)
# see my-app.js function
session$sendCustomMessage("notification", message)
}
Pay attention to the options$icon
element. As we can’t convert Shiny tags to JSON, it
must be converted to character first. If multiple parameters should contain tags, you must treat them accordingly.
25.3 Initialize the widget
On the JS side, we create a new script, widgets.js
:
We set an array containing all compatible widget names in two categories and concatenate
in a widgets
element:
const uiWidgets = ['gauge', 'swiper', 'searchbar'];
const serverWidgets = ['toast', 'photoBrowser', 'notification'];
const widgets = uiWidgets.concat(serverWidgets);
Notice that as we are going to use the app
object, we import them from the init.js
script, located
in the same ./srcjs
folder.
We then define the activateWidget
function, only considering UI widgets.
const activateWidget = (widget) => {
// function logic
; }
Since we have two widgets categories, this function first checks whether the widget is part of the uiWidgets
array with indexOf
:
const activateWidget = (widget) => {
if (uiWidgets.indexOf(widget) > -1) {
// Init widget
}; }
As there may be multiple widgets of the same type, we must loop through all
possible elements. This is where the class is important and must match the widget generic name.
For instance, the gauge has the gauge
class, and the methods are always app.gauge.
.
How do we loop through multiple widgets? We use the jQuery each
method:
const activateWidget = (widget) => {
if (uiWidgets.indexOf(widget) > -1) {
$('.' + widget).each(function() {
// Init widget
}
}; }
We see that $('.' + widget)
gives $('.gauge')
when the widget is a gauge, which
targets all gauges one by one. Then for each gauge, we extract the configuration containing
all options passed by the end user. Remember that each element has a unique id.
We extract the current element $(this)
in the $el
variable and search for a
script tag pointing to the unique tag having $el.attr('id')
as id. The configuration is parsed
and converted to an object. Note that most of the time, Framework7 expects to have el
attributes which simply contain the CSS selector of the current element,
in other words its unique id '#' + $el.attr('id')
:
let $el = $(this);
let config = $(document).find(
'script[data-for="' + $el.attr('id') + '"]'
;
)= JSON.parse(config.html());
config // add the id
.el = '#' + $el.attr('id'); config
The final step consists of initializing the widget, which is quite straightforward if we notice that
app.gauge
is the same as app["gauge"]
. We obtain the general code:
.create(config); app[widget]
For the server widgets, it is even simpler. We recover the message with a Shiny.addCustomMessageHandler("type", callback)
and
initialize it. The only possible source of problem is the custom message type
that must be the same
as the one specified in the R function with session$sendCustomMessage("type", message)
. As shown in the below code, we can chain methods and immediately open the widget, right after its creation. Moreover, it is always good practice to let Shiny know about the widget state, that is whether it is currently opened. This is the reason why we added an on
property to the message. All widgets trigger events,
for instance, notifications have the notification:opened
and notification:closed
. For each event, we set an input value on the fly, with Shiny.setInputValue
as explained in section 12.3. This way, our future users can know exactly when the widget is closed or opened, thereby being able to trigger any subsequent action. This obviously requires the widget to pass an optional id
attribute to ensure the uniqueness.
// Server widget logic
.addCustomMessageHandler(widget, function(message) {
Shinyif (message.id !== undefined) {
.on = {
messageopened: function() {
.setInputValue(message.id, true);
Shiny,
}closed: function() {
.setInputValue(message.id, false);
Shiny
};
}
}
.create(message).open();
app[widget]; })
We create an else
statement following the if
condition (handling the UI widgets) and put the server widget logic inside:
const activateWidget = (widget) => {
if (uiWidgets.indexOf(widget) > -1) {
// UI widget logic
else {
} // Server widget logic
}; }
The full JavaScript code may be found here.
The final step aims at activating all widgets. We proceed with a forEach
loop:
// Loop over all widgets to activate them
.forEach(function(w) {
widgetsactivateWidget(w);
; })
Let’s try below with a notification example, where we capture the state of the notification in an input element. This triggers another notification on the server side, in another observeEvent()
.
### RUN ###
# OSUICode::run_example(
# "shinyMobile/notification",
# package = "OSUICode"
# )
### APP CODE ###
library(shiny)
library(OSUICode)
ui <- f7_page(
allowPWA = FALSE,
navbar = f7_navbar("Notifications"),
toolbar = f7_toolbar(),
title = "Notifications"
)
server <- function(input, output, session) {
observeEvent(TRUE, once = TRUE, {
f7_notif(
id = "welcome",
"Helloooooo",
options = list(closeTimeout = 2000)
)
})
observeEvent(input$welcome, {
showNotification(sprintf("Notification is %s", input$welcome))
})
}
shinyApp(ui, server)
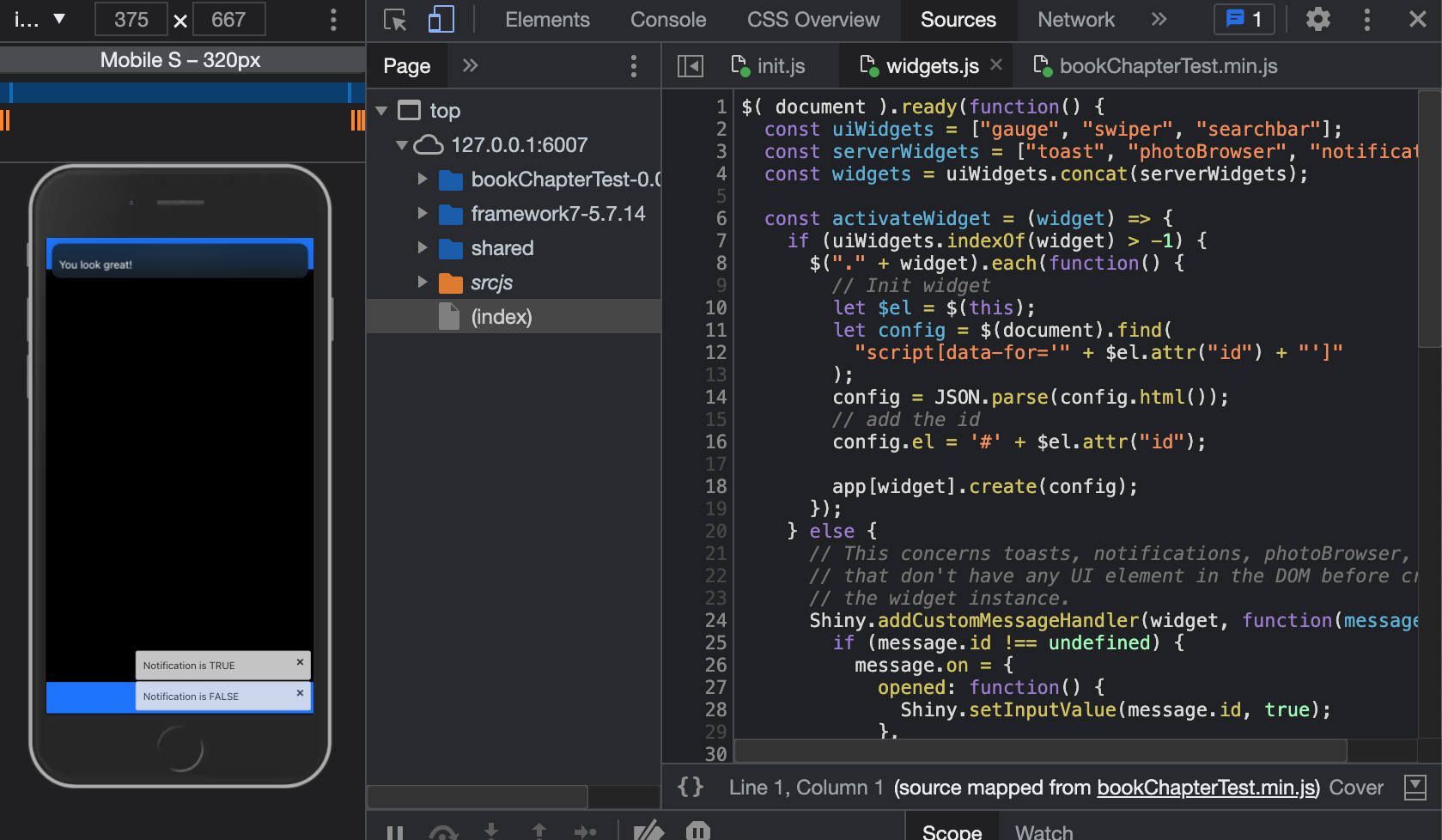
FIGURE 25.2: Notification widget (top).
25.4 Update widgets
We would like to develop a similar generalized interface to update any element in the DOM. Instead of having a collection of function like update_f7_gauge()
or update_f7_swiper()
, we want a single update_f7_instance()
function, which will be easier to maintain.
We leverage the app.data
element (see Chapter 23.5.6) to store all instances by widget type. Inside helper_config.js
, we add a empty gauge array to config.data
:
.data = function() {
configreturn {
// any other widget type to cache ...
gauge: []
;
}; }
The array name must be the same as the app method. For instance,
we have app.gauge
, which means that we should create config.data.gauge
and not config.data.gauges
, as it would lead to errors later.
Once the cache is available, we have to modify the JavaScript, which creates the widget instance,
to store the new instance in the cache, as shown Figure 25.3. We add the following code, where w
refers to the widget instance:
// ui widgets
.data[widget][$el.attr('id')] = w; app
This manipulation does not make sense for server widgets as they are already generated by the server.
The activateWidget
function should be:
// Instantiate a widget
const activateWidget = (widget) => {
// Handle ui side widgets
if (uiWidgets.indexOf(widget) > -1) {
$('.' + widget).each(function() {
// unchanged
// feed the create method
let w = app[widget].create(config);
// Store the widget instance in the app data cache
.data[widget][$el.attr('id')] = w;
app;
})else {
} // Server widgets logic
// Unchanged
}; }
Once done, this is time to design update_f7_instance()
. The R code sends a message to
the current session containing:
- The id of the element to update.
- The new configuration.
Since we send a JSON, the hardest part is to correctly process shiny tags. How do we track shiny tags? As a reminder, let’s run the code below:
#> [1] "shiny.tag"
#> [1] "shiny.tag.list" "list"
For each configuration element, we must check whether its class contains shiny.tag
or shiny.tag.list
and convert it to a character. Moreover, it may contain a nested list, like this:
options = list(
buttons = list(
list(
text = "Some text",
icon = f7Icon("info"),
color = "pink"
)
)
)
In that case, our function must be recursive to handle any item having the list
class. If the element is simple text or numeric, we return it as is.
We finally get:
update_f7_instance <- function(
id,
options,
session = shiny::getDefaultReactiveDomain()
) {
# Convert any shiny tag into character so that toJSON
# does not cry
listRenderTags <- function(l) {
lapply(
X = l,
function(x) {
if (inherits(x, c("shiny.tag", "shiny.tag.list"))) {
as.character(x)
} else if (inherits(x, "list")) {
# Recursive part
listRenderTags(x)
} else {
x
}
}
)
}
options <- listRenderTags(options)
message <- list(id = session$ns(id), options = options)
session$sendCustomMessage("update-instance", message)
}
On the JS side, we receive the message, still in the widget.js
script:
.addCustomMessageHandler(
Shiny'update-instance',
function(message) {
// Treat message ...
}; )
All widgets are stored by type in the app data, for instance, the element having a unique id
mygauge
is located in app.data['gauge']['mygauge']
. As there is no easy way to recover the widget type given its id, the first step of the message handler is to find where our instance is located. We design a nested for loop. The outer loop scans all app.data
properties (i.e. widget categories), while the inner loop scans all existing instances for each category. Whenever, the message.id
matches the instance name, we store the corresponding widget category in a variable:
let instanceFamily;
for (const property in app.data) {
for (const e in app.data[property]) {
if (e === message.id) {
= property;
instanceFamily
}
} }
We then access the old instance using the newly defined variable and the message.id
.
We capture its parameters located in oldInstance.params
. From there, multiple options are available:
- We extend the old configuration with the new one.
- We entirely overwrite the existing options.
In what follows, we decided to merge the old and new configurations using app.utils.extend
:
let oldInstance = app.data[instanceFamily][message.id];
let oldConfig = oldInstance.params;
let newConfig = app.utils.extend(oldConfig, message.options);
The next steps consist of destroying the old instance, initializing the new instance, and refreshing
the app.data
cache:
// Destroy old instance
.destroy();
oldInstance// Create new config
let newInstance = app[instanceFamily].create(newConfig);
// Update app data
.data[instanceFamily][message.id] = newInstance; app
The whole code can be found here. The update concept is illustrated Figure 25.3.
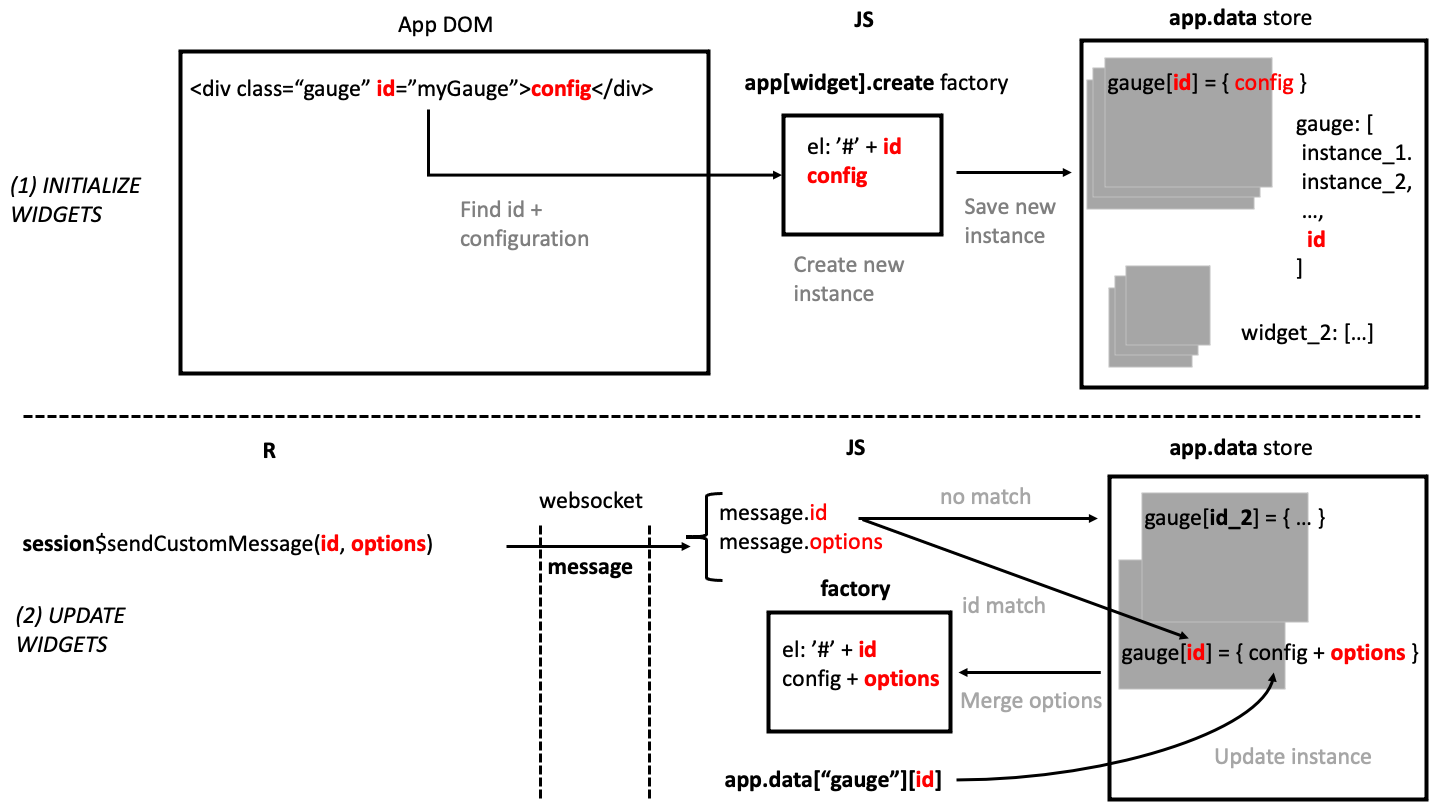
FIGURE 25.3: Initializing and updating widgets in the app.data store.
The code below is an example showing how to update a gauge from the server (Figure 25.4). As you may notice, this
approach is not perfect as the user has to explicitly update the valueText
field so that
it reflects the new value. Similarly, you may ask why the gauge value has to be between 0 and 1,
instead of 0 and 100. The reason comes from the Framework7 API. You might be tempted to convert the value inside f7_gauge()
(so that the user only provides a number between 0 and 100), but
this would also mean having to manually convert the value in the update_f7_instance()
function later. As stated in previous chapters, there is always a compromise between a simple API that is easy for the developer to maintain and the user’s experience. This issue may/should be solved with comprehensive documentation.
### RUN ###
# OSUICode::run_example(
# "shinyMobile/update-gauge",
# package = "OSUICode"
# )
### APP CODE ###
library(shiny)
library(OSUICode)
ui <- f7_page(
allowPWA = FALSE,
div(
class = "block",
f7_gauge(
"mygauge",
value = 0.1,
options = list(
type = "semicircle",
borderColor = "#2196f3",
borderWidth = 10,
valueFontSize = 41,
valueTextColor = "#2196f3",
labelText = "amount of something"
)
)
),
navbar = f7_navbar("Update gauge instance"),
toolbar = f7_toolbar(),
title = "shinyMobile"
)
server <- function(input, output, session) {
observeEvent(TRUE, once = TRUE, {
Sys.sleep(2)
update_f7_instance(
"mygauge",
options = list(
value = 0.75,
valueText = "75 %",
labelText = "New label!"
)
)
})
}
shinyApp(ui, server)
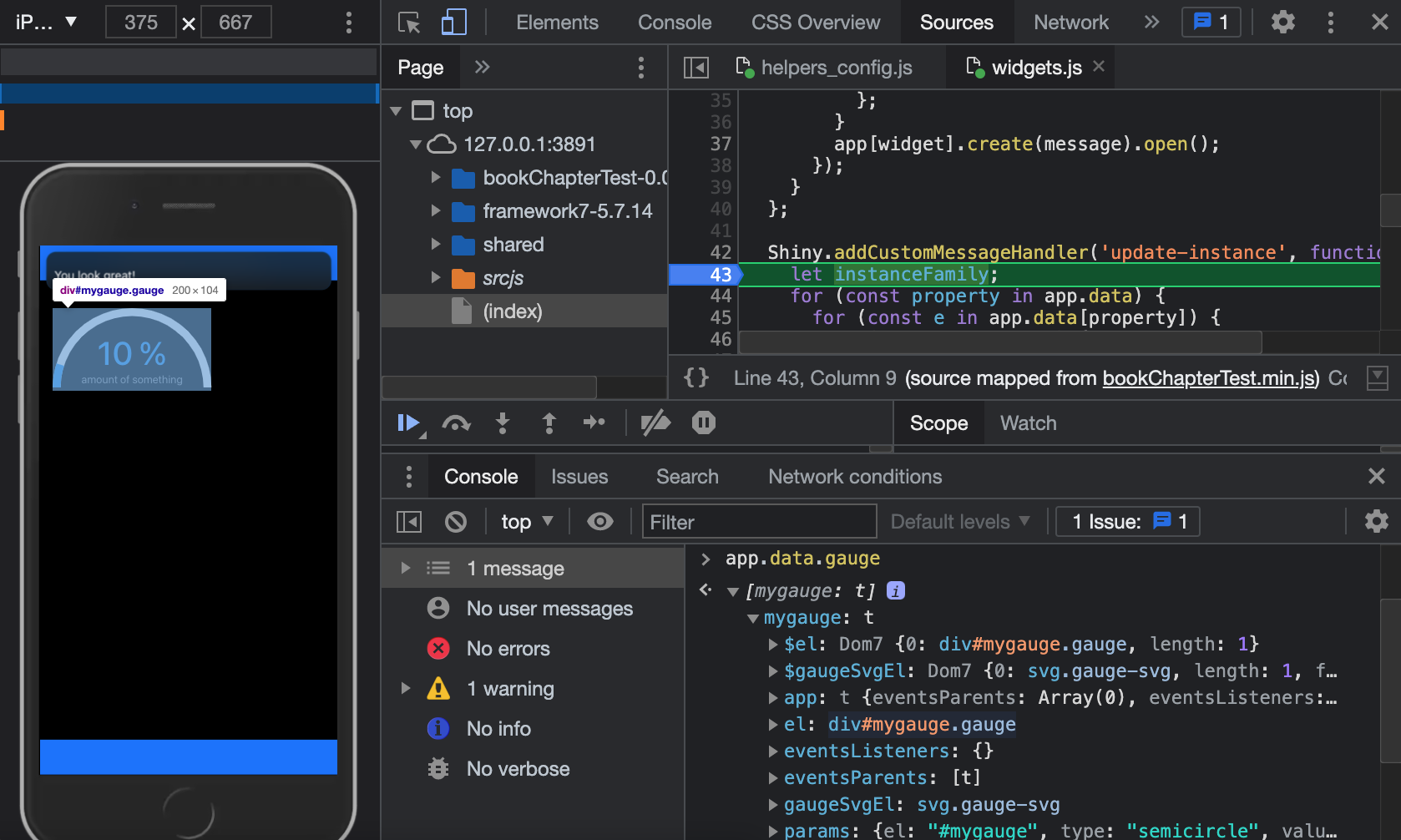
FIGURE 25.4: Update gauge on the server side. We inspect app.data.gauge within the JS console.
If you don’t see the Install
button, copy over the www
folder from your PWA app.
25.5 More complex elements
We consider the tooltip
example. A tooltip is a help text generally displayed on hover (or click) over a specific element. They are commonly used to improve user experience in all websites. Framework7 provides two tooltips APIs:
- A purely-UI side API where the tooltip is attached to a tag.
- A server-side API where the tooltip is dynamically injected in the page content.
While the first approach is obviously not interesting for us since it does not involve a single line of JS, the second approach heavily relies on the app.data
object. This is the one we choose to study.
25.5.1 Add a tooltip
In the following, we design the add_f7_tooltip()
function that may be added right after the existing gauge and notification widgets. Since a tooltip is attached to a DOM element, add_f7_tooltip()
must provide parameters to identify its target, that is either id or CSS selector, but not both. Therefore, we create a helper function, validate_selector()
, that checks if the end user provides id
or selector
.
validate_selector <- function(id, selector) {
if (!is.null(id) && !is.null(selector)) {
stop("Please choose either target or selector!")
}
}
%OR%
ensures that we return a
if not NULL
otherwise b
.
"%OR%" <- function(a, b) if (!is.null(a)) a else b
add_f7_tooltip()
sends the tooltip target, as well a list of options to JS with the add_tooltip
identifier.
add_f7_tooltip <- function(
id = NULL,
selector = NULL,
options,
session = shiny::getDefaultReactiveDomain()
) {
# We use already defined popover functions
validate_selector(id, selector)
if (!is.null(id)) id <- paste0("#", session$ns(id))
options$targetEl <- id %OR% selector
session$sendCustomMessage("add_tooltip", options)
}
On the JS side within widgets.js
, we recover the message by building a custom message handler with Shiny.addCustomMessageHandler
pointing to add_tooltip
. Again, if there is a mismatch between R and JS type, the API won’t work. Like all Framework7 widgets,
creating a tooltip is rather straightforward:
.addCustomMessageHandler('add_tooltip', function(message) {
Shiny.tooltip.create(message).show();
app; })
As mentioned earlier, this syntax is fine since message
is a JSON element and we can also chain methods. There is however a problem: add_tooltip
is fired
each time the user triggers a specific element on the R side. It means the tooltip instance is
created each time, which is not optimal. To fix this issue, we set a tooltips cache in the app.data
object and accordingly modify helpers_config.js
:
.data = function() {
configreturn {
gauge: [],
tooltips: []
;
}; }
We update the previous custom handler so that we:
- Check if the tooltip instance is already in the cached
app.data
before creating an instance. If is is already there, nothing has to be done. - Each time we create a new instance, we save it in the
app.data
cache to retrieve it later.
.addCustomMessageHandler('add_tooltip', function(message) {
Shiny// We store all created instances in app data so that we don't
// recreate them later if they exist ...
if (app.data.tooltips[message.targetEl] === undefined) {
// create instance
let t = app.tooltip.create(message);
// Open tooltip
.show();
t// Storage in app data (tooltips array)
.data.tooltips[message.targetEl] = t;
app
}; })
We store the current instance with app.data.tooltips[message.targetEl] = t;
.
The reference is given by message.targetEl
that is the target to apply the tooltip on. When multiple tooltips are created, we may search for them by target name, which is a reasonable choice. For instance, app.data.tooltips["#mybutton"]
points to the tooltip associated with the element having #mybutton
as id. So far so good!
### RUN ###
# OSUICode::run_example(
# "shinyMobile/add-tooltip",
# package = "OSUICode"
# )
### APP CODE ###
library(shiny)
library(OSUICode)
shinyMobile_options$dark <- FALSE
ui <- f7_page(
allowPWA = FALSE,
options = shinyMobile_options,
tags$div(
class = "block",
tags$button(
id = "mybutton",
class = "button button-large button-fill",
"Click"
)
),
navbar = f7_navbar("Add tooltip"),
toolbar = f7_toolbar(),
title = "Notifications"
)
server <- function(input, output, session) {
observeEvent(TRUE, once = TRUE, {
add_f7_tooltip(
id = "mybutton",
options = list(text = "This is a button")
)
})
}
shinyApp(ui, server)
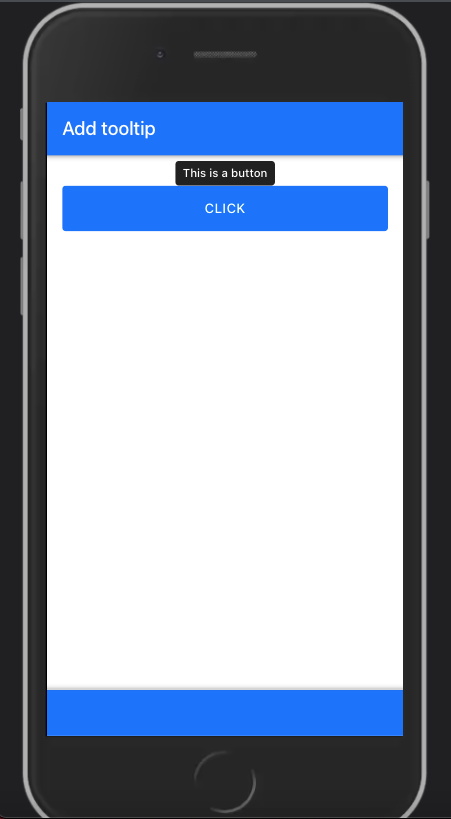
FIGURE 25.5: Create a tooltip on the server and attach it to a DOM element.
25.5.2 Update a tooltip
Framework7 does not provide any native enable/disable
method, which means that once the tooltip is activated on a given element, it is visible forever (Figure 25.5), unless destroyed.
The main purpose of update_f7_tooltip()
is to avoid this, by temporarily disabling any tooltip and reactivating it later. Like for add_f7_tooltip()
, we may target either by id
or provide a more complex CSS selector. We support two actions: toggle the tooltip (enable/disable) and update, that is, change data like the displayed text.
update_f7_tooltip <- function(
id = NULL,
selector = NULL,
action = c("toggle", "update"),
text = NULL,
session = shiny::getDefaultReactiveDomain()
) {
validate_selector(id, selector)
if (!is.null(id)) id <- paste0("#", session$ns(id))
targetEl <- id %OR% selector
message <- dropNulls(
list(
targetEl = targetEl,
action = action,
text = text
)
)
session$sendCustomMessage("update_tooltip", message)
}
update_f_tooltip()
sends three elements to JS, namely the tooltip target element message.targetEl
, the optional new text, that is message.text
and the action to perform message.action
(either update or toggle state).
The corresponding JS handler:
- Checks if the specified tooltip instance exists and only update if it is the case.
- Handles two situations: updating the tooltip content or toggling the tooltip visibility. It is actually more than just showing/hiding the tooltip. Remember that each tooltip is shown on hover so applying hide on a visible tooltip will only have effect until the user hovers again over the same tooltip, which is useless.
In widgets.js
, right after the add_tooltip
handler, we add the update
logic, that consists in changing the text:
.addCustomMessageHandler(
Shiny'update_tooltip', function(message) {
// Don't do anything if the instance is not there in app data
if (app.data.tooltips[message.targetEl] !== undefined) {
let t = app.data.tooltips[message.targetEl];
if (message.action === 'update') {
.setText(message.text);
t
}
}; })
The corresponding tooltip instance is accessed in app.data
with app.data.tooltips[message.targetEl]
and stored in a local variable, t
. We apply the Framework7 tooltip method setText
only if the user action corresponds to update
.
The next step is to handle the toggle
case. We check whether the current instance is active, applying the app.tooltip.get
method on message.targetEl
. If the instance is alive, we get an object, while we obtain undefined if it does not exist. We then call the app.tooltip.destroy
method on the current instance:
.addCustomMessageHandler(
Shiny'update_tooltip', function(message) {
// Don't do anything if the instance is not there in app data
if (app.data.tooltips[message.targetEl] !== undefined) {
let t = app.tooltip.get(message.targetEl);
if (message.action === 'update') {
.setText(message.text);
telse if (message.action === 'toggle') {
} // destroy
if (t) {
.destroy();
t
}
}
}; })
Wait a moment … There are two issues with this code. As t
is a variable pointing
to the current instance, if we destroy that instance, t
will point to a destroyed element the next time it is called, and any action like setText
will raise an error. The trick is to create a shallow clone with Object.assign
that won’t be affected by the destroy
method, save the shallow clone in the app.data
cache and destroy the old instance. Therefore, we update the tooltips.js
script:
.addCustomMessageHandler(
Shiny'update_tooltip', function(message) {
if (app.data.tooltips[message.targetEl] !== undefined) {
// Try to get the instance
let t = app.tooltip.get(message.targetEl);
if (message.action === 'update') {
if (t) {
.setText(message.text);
t
}else if (message.action === 'toggle') {
} if (t) {
// create copy that won't be modified if
// t is destroyed!
let cachedTooltip = Object.assign({}, t);
// save copy to replace the deleted one in the app data
.data.tooltips[message.targetEl] = cachedTooltip;
app// destroy current instance
.destroy();
t
}
}
}; })
We also check whether the instance is alive before updating it. We are still missing the re-activation
step that consists in rebuilding the tooltip instance based on the cached data app.data.tooltips[message.targetEl]
previously saved. All parameters are contained in the params
object (instance element):
// Capture parameters
let pars = app.data.tooltips[message.targetEl].params;
// Recreate the tooltip based on the copy configuration
= app.tooltip.create(pars);
t // Replace the app data instance
.data.tooltips[message.targetEl] = t; app
The final JS code is:
.addCustomMessageHandler(
Shiny'update_tooltip', function(message) {
if (app.data.tooltips[message.targetEl] !== undefined) {
// Unchanged
if (message.action === 'update') {
// Unchanged
else if (message.action === 'toggle') {
} if (t) {
// Unchanged
else {
} // Capture parameters
let pars = app.data.tooltips[message.targetEl].params;
// Recreate the tooltip based on the copy configuration
= app.tooltip.create(pars);
t // Replace the app data instance
.data.tooltips[message.targetEl] = t;
app
}
}
}; })
You may find an example below.
### RUN ###
# OSUICode::run_example(
# "shinyMobile/update-tooltip",
# package = "OSUICode"
# )
### APP CODE ###
library(shiny)
library(OSUICode)
shinyMobile_options$dark <- FALSE
ui <- f7_page(
allowPWA = FALSE,
options = shinyMobile_options,
tags$div(
class = "block",
tags$button(
id = "mybutton",
class = "button button-large button-fill action-button",
"Click"
),
tags$label(
class = "toggle",
tags$input(type = "checkbox", id = "enable"),
tags$span(class = "toggle-icon")
)
),
navbar = f7_navbar("Add tooltip"),
toolbar = f7_toolbar(),
title = "Notifications"
)
server <- function(input, output, session) {
observeEvent(TRUE, once = TRUE, {
add_f7_tooltip(
id = "mybutton",
options = list(text = "This is a button")
)
})
observeEvent(input$enable, {
update_f7_tooltip(
id = "mybutton",
action = "toggle"
)
})
observeEvent(input$mybutton, {
update_f7_tooltip(
id = "mybutton",
action = "update",
text = "New tooltip text"
)
})
}
shinyApp(ui, server)